Get a token for public apps
Your app will receive a short-lived access token (24 hrs) when you register an event in the setup steps or webhook events. You can use this access token to call the Envoy API to perform an action. However, sometimes you may need to call the API without relying on an event from Envoy or you may need an access token for longer than 24 hours.
In those instances, you can request a new access token whenever your app is installed by a user. This will provide you with a refresh token too, so that you can refresh the token automatically when it expires.
1. Create a setup step and add a validation URL.
Whenever a user reaches this step while installing your app Envoy will send a route webhook containing an access token.
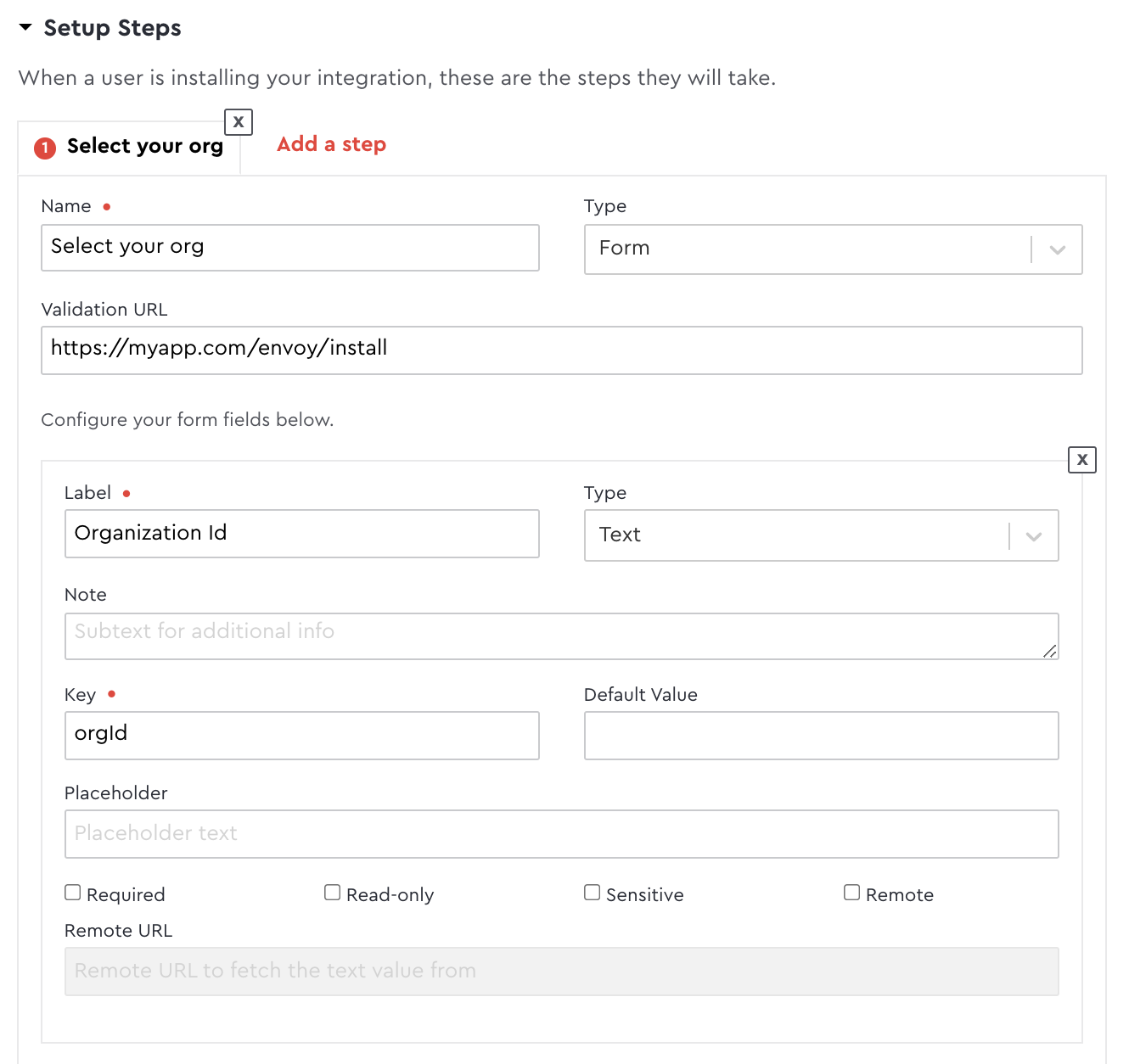
2. Use the access token from the route webhook to get an access token for user's install.
The route webhook will contain an auth object with an access token and an install id.
{
"name": "route",
"request_meta": {
"auth": {
"state": null
"company_id": "100000",
"expires_in": 86400,
"token_type": "Bearer",
"access_token": "ACCESS_TOKEN",
"refresh_token": null,
"refresh_token_expires_in": null
},
"route": "step-0-field-1-options",
"plugin_id": "PLUGIN_ID",
"install_id": 1000000
}
}
3. Call the token API to get a new access token and a refresh token
Using the install id from the route webhook you can request a new access token and get a refresh token.
curl --location --request POST 'https://api.envoy.com/oauth2/token' \
--form 'client_id=[CLIENT_ID]' \
--form 'client_secret=[CLIENT_SECRET]' \
--form 'install_id={INSTALL_ID}' \
--form 'grant_type=plugin_install'
{
"token_type": "Bearer",
"access_token": "{YOUR_ACCESS_TOKEN}",
"expires_in": 86400,
"refresh_token": "{YOUR_REFRESH_TOKEN}",
"refresh_token_expires_in": 2592000,
"state": null,
"company_id": "10000"
}
4. Exchange your refresh token for a new access token
When your access token expires you can receive a new one by using the refresh token.
curl --location --request POST 'https://api.envoy.com/oauth2/token' \
--form 'client_id={ENVOY_CLIENT_ID}' \
--form 'client_secret={ENVOY_CLIENT_SECRET}' \
--form 'refresh_token={REFRESH_TOKEN}' \
--form 'grant_type=refresh_token'
{
"token_type": "Bearer",
"access_token": "{YOUR_ACCESS_TOKEN}",
"expires_in": 86400,
"refresh_token": "{YOUR_REFRESH_TOKEN}",
"refresh_token_expires_in": 2592000,
"state": null,
"company_id": "10000"
}
Updated over 3 years ago