Make your first API request
Learn how to get started using the Envoy API.
This tutorial describes the basic steps for getting started with the Envoy API. This tutorial will show you how to get an access token and then call the locations API to fetch details about your Envoy location(s).
Create a developer account
Use the Dev Dashboard to create and manage apps for use privately within your company or to share publicly with others.
Create an API user
Before you can make a call to the API, you need to set up an API user account. For demonstration purposes, this guide will show you how to authenticate with the Envoy API using the OAuth2 password grant type.
The password grant type should only be used for private apps you are building for just your company. If you are building apps for others, you'll need to authenticate by following this guide.
- Create an employee manually in your employee directory.
- Assign the user a Global Admin role.
- Make sure the setting Show employee as a host on the Visitors Kiosk is disabled
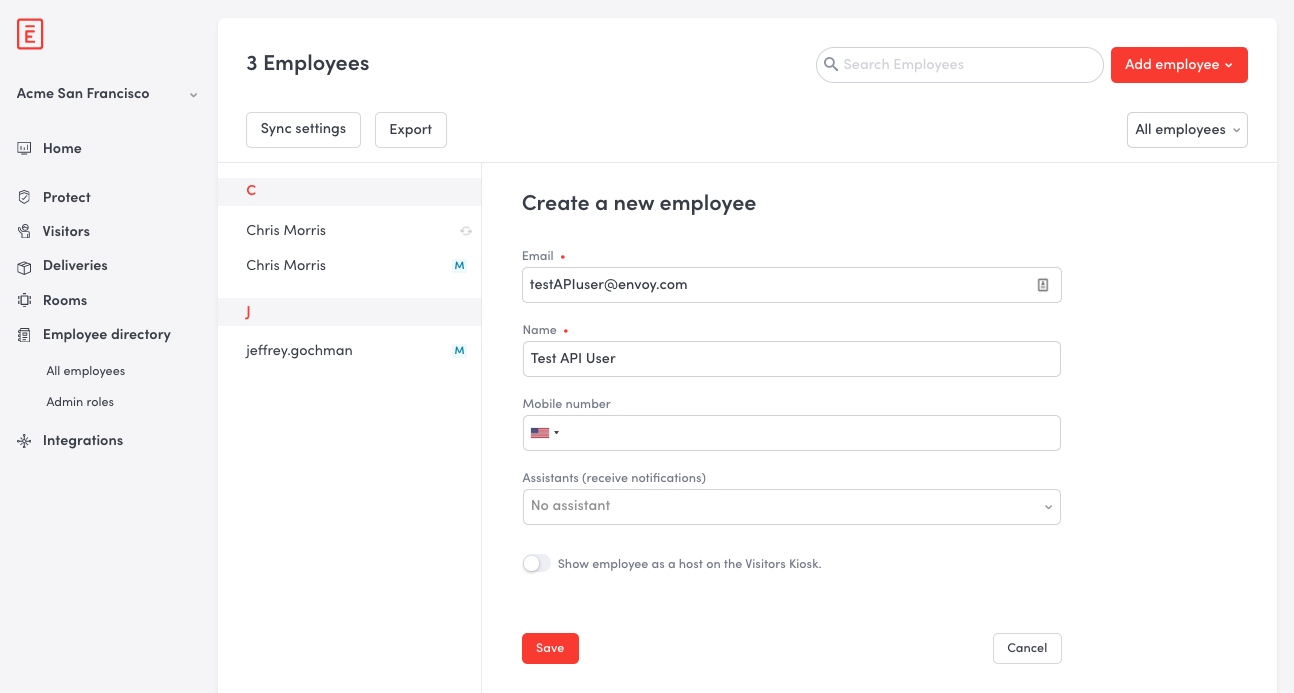
Get a client id and secret
You'll need a client id and secret to retrieve an access token to authenticate with the API. To generate a client id and secret you will need to create an integration using the Dev Dashboard.
- Go to the Dev Dashboard.
- Click Create New.
- Provide a name for your integration
- Under API Scopes add the scope
locations.read
- Check Is the integration ready to be displayed?
- Click Save Integration
Here's what your integration configuration settings should look like
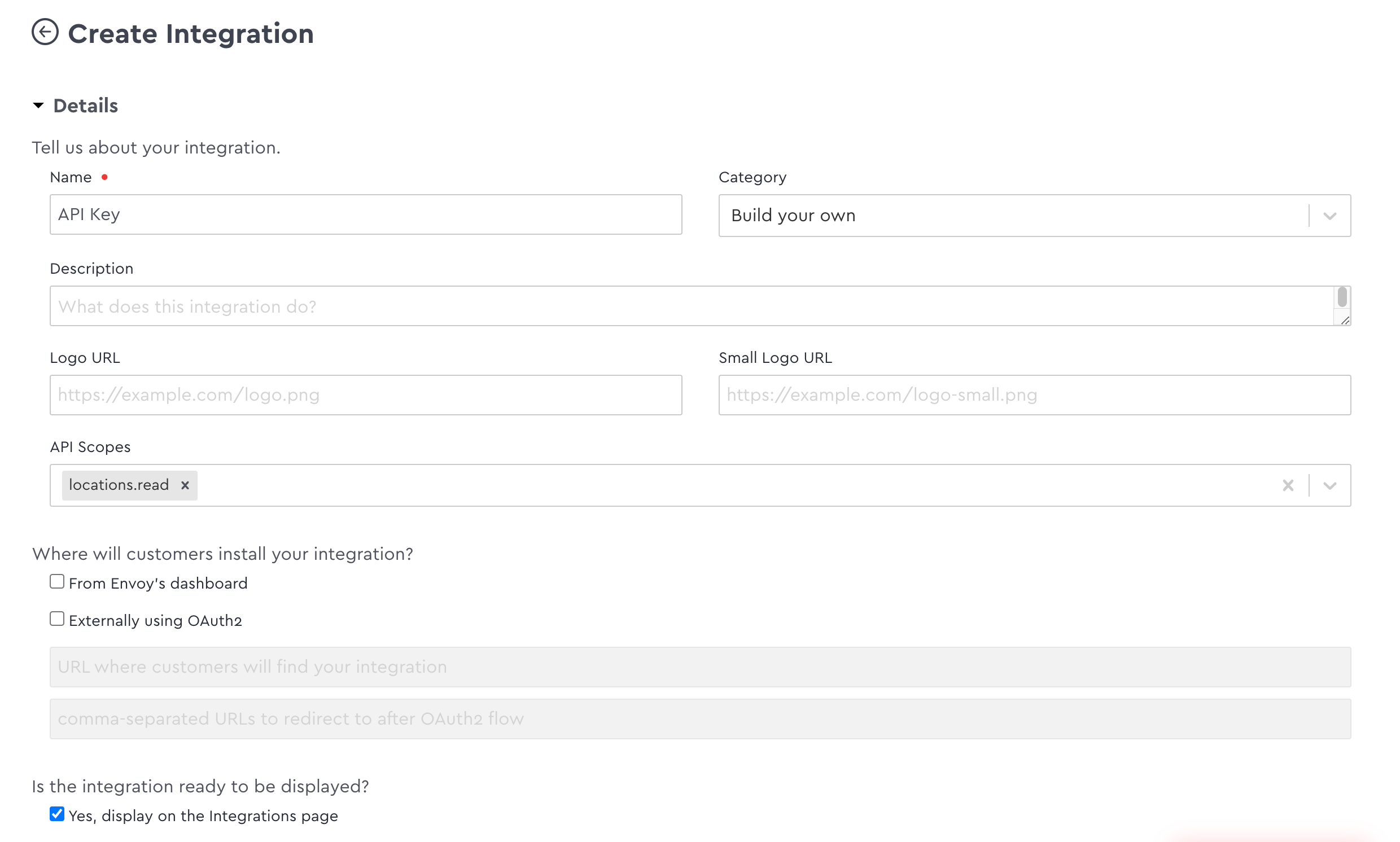
- From the All Integrations tab within the Dev Dashboard find the integration you just created and select Configure.
- Copy the client id and client secret from the Envoy API Credentials section.
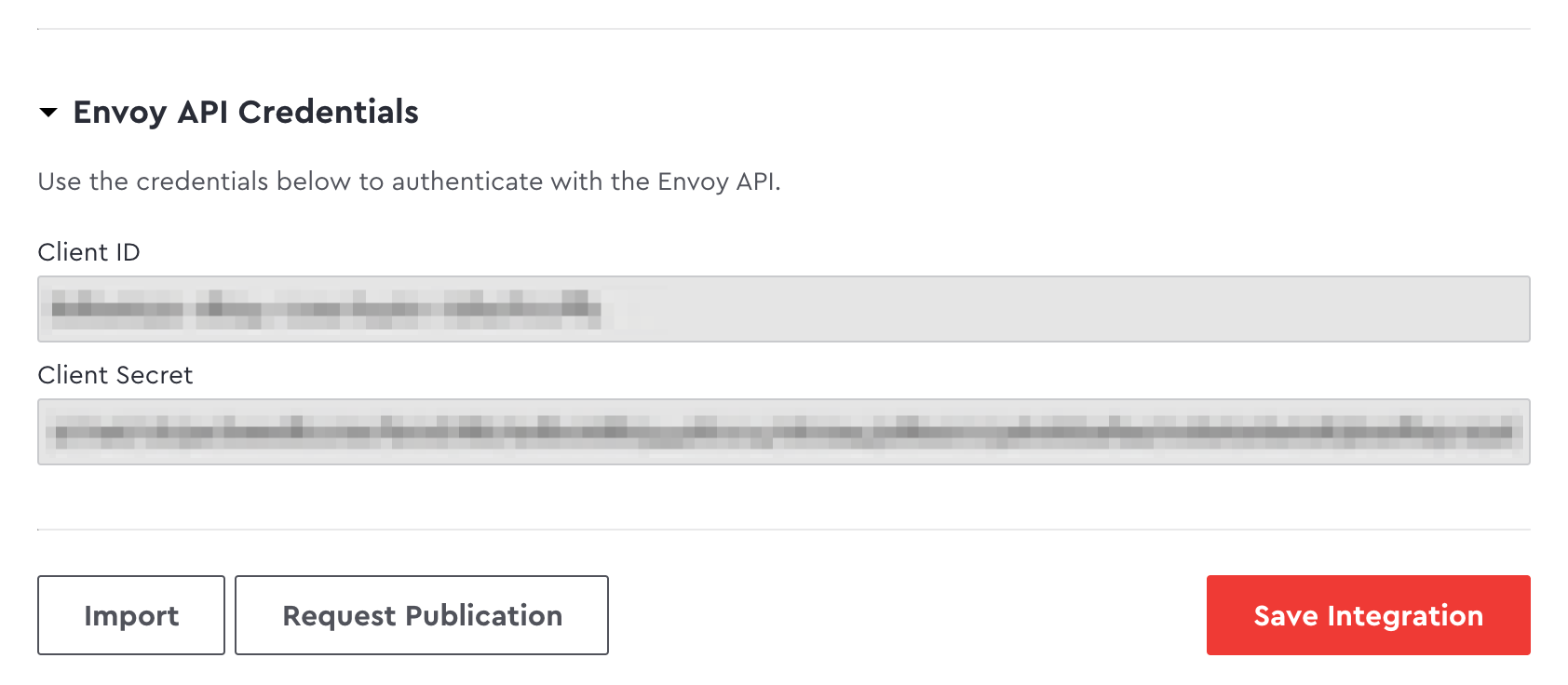
Get an access token
Next you'll need to exchange the client id, secret, and username and password to get an access token that can be used to authenticate your API request.
Call the token API oauth2/token
and format your request using the curl example below.
curl --location --request POST 'https://api.envoy.com/oauth2/token' \
--header "Authorization: Basic $( echo -n {ENVOY_CLIENT_ID}:{ENVOY_CLIENT_SECRET} | base64 )" \
--form "username={USER_EMAIL}" \
--form "password={PASSWORD}" \
--form "scope=token.refresh,locations.read" \
--form "grant_type=password"
{
"token_type": "Bearer",
"access_token": "{YOUR_ACCESS_TOKEN}",
"expires_in": 86400,
"refresh_token": "{YOUR_REFRESH_TOKEN}",
"refresh_token_expires_in": 2592000,
"state": null,
"company_id": "10000"
}
Remember to base64 encode your client ID and secret in the authorization header.
Name | Description |
---|---|
authorization | Basic auth header using the client ID and secret as the username and password base64 encoded. |
client_id | The client ID for your application. |
client_secret | The client secret for your application. |
grant_type | Set to password . |
scope | Set to token.refresh,locations.read . This will allow us to retrieve location information. If you want to fetch other types of data you'll need to include the appropriate scopes. Learn more about scopes. |
Make an API request
To get a list of locations for your account, use the access token that was returned in the previous step in the authorization header and call the locations API /api/v1/locations
.
curl --location --request GET 'https://api.envoy.com/rest/v1/locations' --header 'Accept: application/vnd.api+json' --header 'Authorization: Bearer {YOUR_ACCESS_TOKEN}'
{
"data": [
{
"id": "94082",
"type": "locations",
"links": {
"self": "https://app.envoy.com/api/v3/locations/94082"
},
"attributes": {
"address": "410 Townsend St 4th floor, San Francisco, CA 94107, USA",
"address-line-one": "410 Townsend St",
"address-line-two": "4th floor",
"auto-sign-out-at-midnight": false,
"blacklist-filters-csv-upload-status": null,
"city": "San Francisco",
"company-name-override": "Envoy",
"deliveries-onboarding-complete": false,
"disabled": false,
"capacity-limit": 5
}
}
If you want to access other resources through the API remember to add the appropriate scopes in the scopes section of the Dev Dashboard and in the
scope
value in the /tokens endpoint.
Updated about 1 month ago